Facebook apps are very popular due to various reasons. Most of the time these apps are built for entertainment purposes.
When you see an attractive Facebook app you click on it. Once you clicked you can notice that they ask you to login as a Facebook, Twitter or Gmail user. That means by having one of these accounts you can use those apps without any problem. But these application owners, are they related to Facebook? or twitter? No! but then what is the connection between them? How we able to access someone else's application using Facebook account? Does Facebook know about this? You might have these questions in your mind.
Actually these applications use OAuth protocol.
OAuth (Open Authorization) is an open standard for token-based authentication and authorization on the Internet. OAuth, which is pronounced "oh-auth", allows an end user's account information to be used by third-party services, such as Facebook, without exposing the user's password.
In this blog post I am going to explain how to make a simple Facebook app like this using OAuth protocol.
But before that let's see what is happening behind this.
Study this diagram.
What is this token Facebook provides? actually Facebook provides two types of tokens. Access token and refresh token. Access token can be used several times before it gets expired. Once it is expired refresh token is sent to Facebook server in order to receive another access token along with a new refresh token.User can use this access token to get information from Facebook.
Let's start creating the app.
First we should go to facebook developer site and create an app there.
https://developers.facebook.com/
Go to "My Apps" on top right corner and select add new app.
This window will pop up. Enter required details and click "create App ID".
Now click on "Add Platform" on left corner navigation list. You will see something like this. Click on "Get started" in Audience Network.
You will see this area. In here you have to provide redirect URL in valid OAuth redirect URLs.
Facebook will send all his responses to this URL.
In "settings" provide a App Domain and a Website URL. To provide a Website you have to click on "+ Platform" and then click on website.
In Dashboard you can see your app's App ID and App secret.
Now let's see how to use these values ( Redirection point URL, App ID, App secret ) to get resources from Facebook.
Obtain Authorization code from Facebook
For this we have to prepare the URL. This URL contains for elements.When we put these elements together all should be encoded using a URL encoding method. Parameter name, value and encoded value is given below.
1. response_type.
Code
Code
2. client_id
1363180347108907
1363180347108907
3. redirect_uri
http://localhost/team/
http%3A%2F%2Flocalhost%2Fteam%2F
4. scope
public_profile user_posts user_friends user_photos
public_profile%20user_posts%20user_friends%20user_photos
Now combine these values and make the URL.
Enter that URL in the URL bar of your browser and hit enter. Now you will see something like this. This is called as user consent page. In there you can see "Edit this" button. If you click on that you can manage the accessing resources.
Since you are the owner of this App you don't have to worry about privacy. Click on continue.
This page will appear.
This page appear because for real you don't have a project which supports http://localhost/team/.
But check the URL. You can see authorization code is sent to you from Facebook. (highlighted)
http://localhost/team/?code=AQDRsjK348Gmy1upjm7vXVWPA5_n3A64gRs43npMFInR7b3H2-ibuf7s9vMaPnx3uqQt_oT2wx7XeICuIUlR2J-xICsHREiV5RmZ_-tqEPxKZYWfbI9qCtUopJBtLPkvC7KkPlWsshukf2siNYG1oAJTI87cYmNPC5_vhFdJeVAG7jqPu-Wbc1ACrLHMkCvMXXiWryWz0hMOGWMiZfgA8kteKuj0Y18fzL8vI156P1UiOiOr9pAz11OXrEPtga
7bZt4UJzzFJ0V8QJ0rof8Kc2HmKvGoaKpOC6oJBpR09fPo2fRs8umhQ5JMa4pHZwpm7j4nI-t4goKumDxpMMnlHG7R#_=_
Obtain access token
To obtain access token we have to have four parameters.
1. grant_type
Authorization_code
2. client_id
1363180347108907
3. redirect_uri
http://localhost/team/
4. code
AQDRsjK348Gmy1upjm7vXVWPA5_n3A64gRs43npMFInR7b3H2-ibuf7s9vMaPnx3uqQt_oT2wx7XeICuIUlR2J-xICsHREiV5RmZ_-tqEPxKZYWfbI9qCtUopJBtLPkvC7KkPlWsshukf2siNYG1oAJTI87cYmNPC5_vhFdJeVAG7jqPu-Wbc1ACrLHMkCvMXXiWryWz0hMOGWMiZfgA8kteKuj0Y18fzL8vI156P1UiOiOr9pAz11OXrEPtga
7bZt4UJzzFJ0V8QJ0rof8Kc2HmKvGoaKpOC6oJBpR09fPo2fRs8umhQ5JMa4pHZwpm7j4nI-t4goKumDxpMMnlHG7R#_=_
In the HTTP Headers, we need to add the Authorization header with the App credentials.
App ID - 1363180347108907
App secret - c12fb940ca8e67d43d447f364861218b
AppID:App_secret
1363180347108907:c12fb940ca8e67d43d447f364861218b
Now we have to encode this whole value using a base64 encoder.
MTM2MzE4MDM0NzEwODkwNzpjMTJmYjk0MGNhOGU2N2Q0M2Q
0NDdmMzY0ODYxMjE4Yg==
To get the access token we have to specify the token endpoint. In this case it is this url
https://graph.facebook.com/oauth/access_token .
Install RESTClient in your browser.
Give those values and obtain access token.
Retrieve resources using access token
Method - GET
URL - https://graph.facebook.com/v2.8/me?fields=id
Authorization: Bearer <access token value>
This will give user's ID in JSON object format.
Using this ID you can get any information you want.
ex- you can uploaded posts.

Now let's see how to implement an app which can retrieve these information and output to the user.
I used php to develop this app.
Github link - https://github.com/sajith01prasad/Facebook-App---Oauth2.0.git
You have to have Facebook SDK v5 for PHP. (You don't have to download this. I have added in it my project. Check folder "facebook" in my project folder)
https://developers.facebook.com/docs/reference/php
index.php
If you are not logged into Facebook only you will see the index page. Otherwise you will directly go to i.php which shows the results.
This page is the main page where user directs to.
Once the user clicks on "Click Here" button user will direct to i.php .
i.php
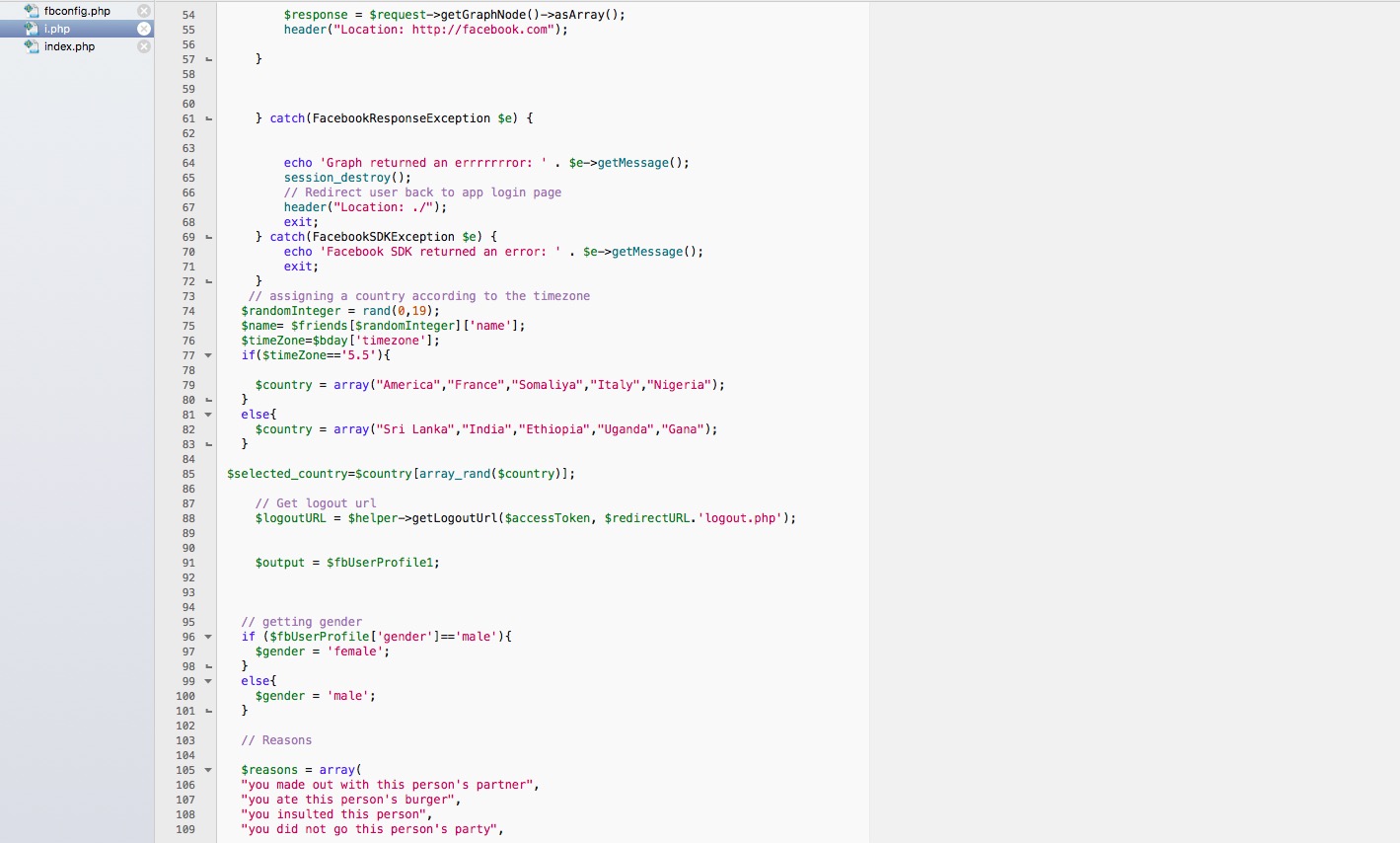
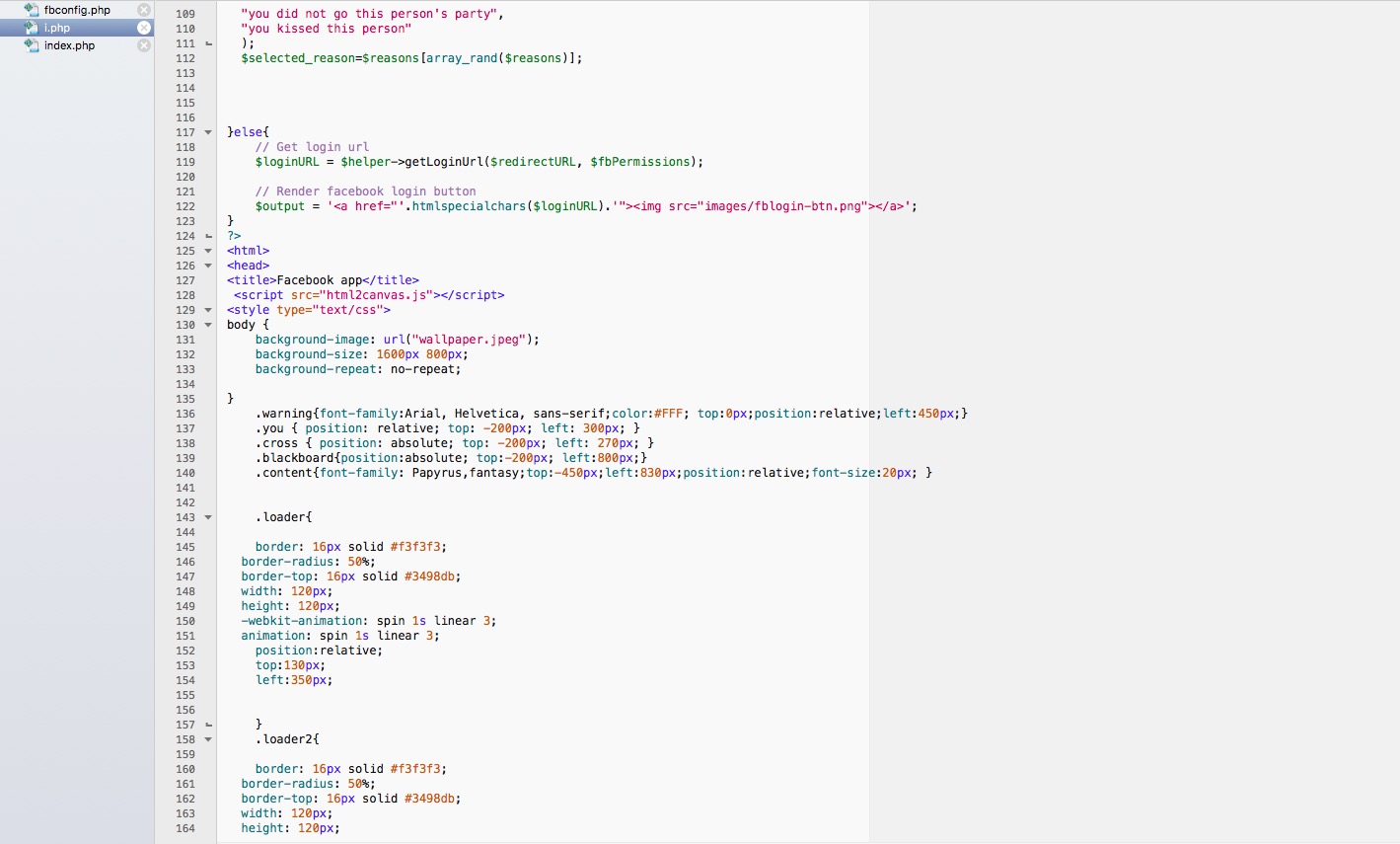

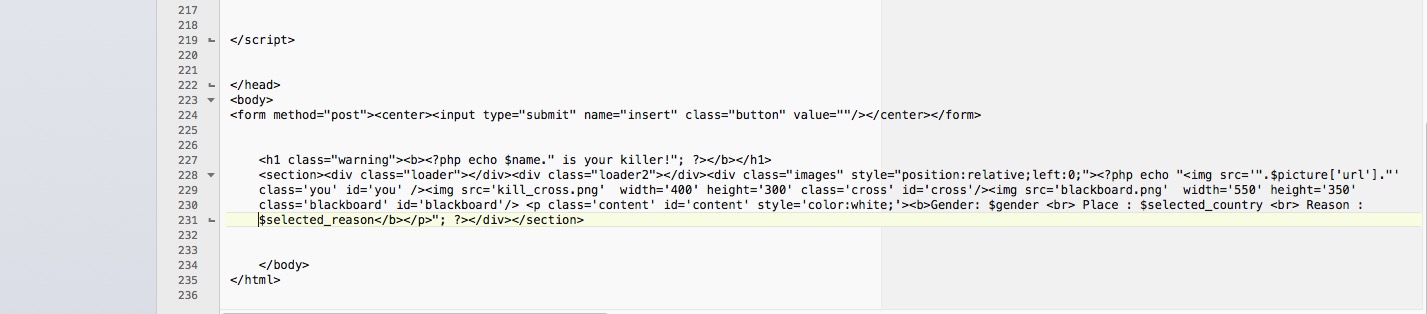
Note:
The app I used for this has permissions for user friendlist. You can go to app review and check which permission you have.

Do not worry. If your app does not have that permission provide this instead of $permissions = ['email']; in both i.php and index.php files
$permissions = array("email","user_friends");
Otherwise you will not get killer's name.
Look of this page is like this.
You can see there is a button on the top of this page "Share on Facebook". Once the user clicks on this button automatically picture of index page with a caption of "Check out this app! It is awesome http://localhost/fb/i.php " will be uploaded on users timeline.
By calling facebook api I have received a few resources of user. name, profile picture, gender, timezone. According to the timezone I am assigning a country to the user. Since my application didn't go under Facebook review process I can't access many user information. (such as birthday, friend's profile picture etc).
These are the files in the facebook php SDK v5
I hope you now you know how to make a Facebook app with the help of OAuth from the scratch.
Cheers. !!!